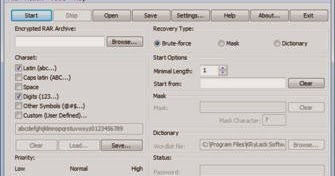
)Q:
Java: Why does this method result in an infinite loop?
While solving a question, I came across the following method. It seemed to result in an infinite loop.
public void exec() {
double num1 = 1;
double num2 = 1;
for (;;) {
if (num1 == 1 && num2 == 1)
break;
num1 = num1 * num2;
num2 = num2 * num1;
}
}
After looking at the solutions, I didn't see what went wrong. The only thing I can think of is that I shouldn't have used 'for (;;)' in this particular case.
Can someone please explain to me why it would result in an infinite loop?
A:
The infinite loop is because of
if (num1 == 1 && num2 == 1)
break;
You break the loop when num1 == 1 && num2 == 1, that's why it never stops!
Here you want to continue the loop when the conditions are fulfilled so you need to remove that break statement
A:
With break the code execution jumps to the next instruction and the statement following it. Without break, it doesn't and the inner loop executes forever.
A:
Your loop is infinite because it always executes the break statement. You do not want that. The line is semantically equivalent to:
double num1 = 1;
double num2 = 1;
while (true) {
num1 = num1 * num2;
num2 = num2 * num1;
}
Now, it is clear that this loops infinitely.
To solve it, make the conditional if into a while-loop:
public void exec() {
double num1 = 1;
double num2 = 1;
while (num1!= 1 || num2!= 1) {
if (num1 == 1 && num2 == 1)
break; ac619d1d87
Related links:
Comments